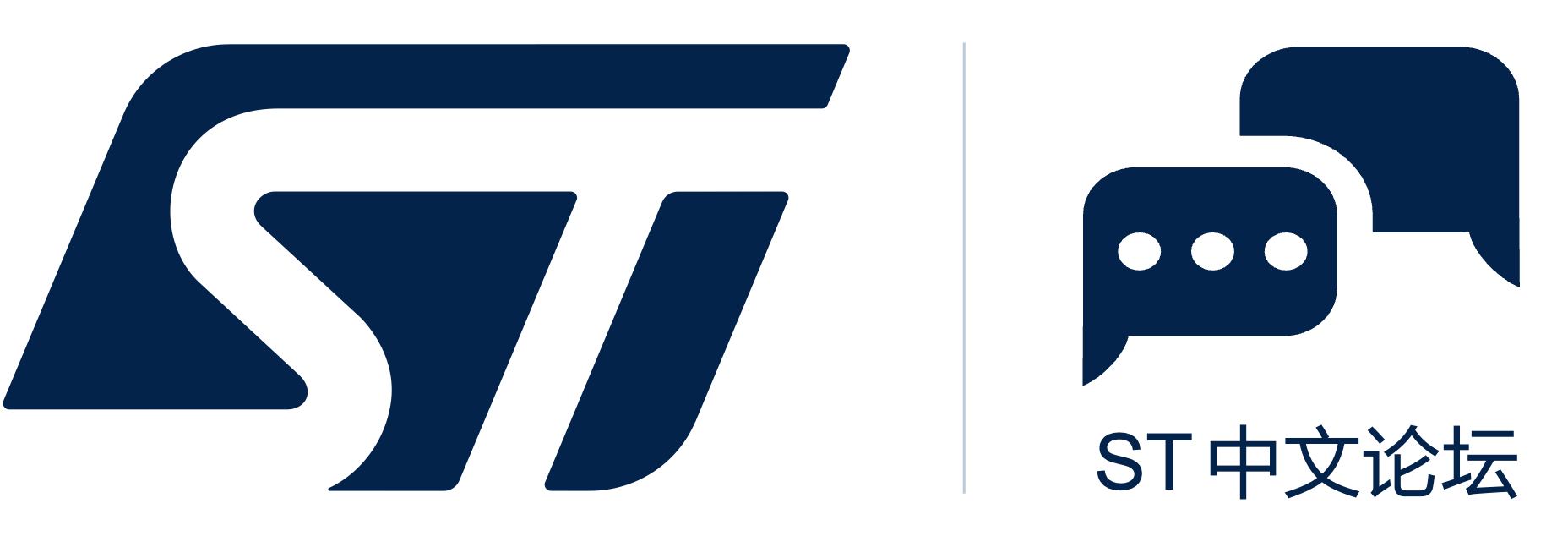
![]() 二里面是产生梯形波,为什么可以产生梯形波?然后产生三角波、等等 重点在HAL_DAC_Start_DMA(&DacHandle, DACx_CHANNEL, (uint32_t *)aEscalator8bit, 6, DAC_ALIGN_8B_R) HAL_StatusTypeDef HAL_DAC_Start_DMA(DAC_HandleTypeDef* hdac, uint32_t Channel, uint32_t* pData, uint32_t Length, uint32_t Alignment) { uint32_t tmpreg = 0; /* Check the parameters */ assert_param(IS_DAC_CHANNEL(Channel)); assert_param(IS_DAC_ALIGN(Alignment)); /* Process locked */ __HAL_LOCK(hdac); /* Change DAC state */ hdac->State = HAL_DAC_STATE_BUSY; if(Channel == DAC_CHANNEL_1) { /* Set the DMA transfer complete callback for channel1 */ hdac->DMA_Handle1->XferCpltCallback = DAC_DMAConvCpltCh1; /* Set the DMA half transfer complete callback for channel1 */ hdac->DMA_Handle1->XferHalfCpltCallback = DAC_DMAHalfConvCpltCh1; /* Set the DMA error callback for channel1 */ hdac->DMA_Handle1->XferErrorCallback = DAC_DMAErrorCh1; /* Enable the selected DAC channel1 DMA request */ hdac->Instance->CR |= DAC_CR_DMAEN1; /* Case of use of channel 1 */ switch(Alignment) { case DAC_ALIGN_12B_R: /* Get DHR12R1 address */ tmpreg = (uint32_t)&hdac->Instance->DHR12R1; break; case DAC_ALIGN_12B_L: /* Get DHR12L1 address */ tmpreg = (uint32_t)&hdac->Instance->DHR12L1; break; case DAC_ALIGN_8B_R: /* Get DHR8R1 address */ tmpreg = (uint32_t)&hdac->Instance->DHR8R1; break; default: break; } } else { /* Set the DMA transfer complete callback for channel2 */ hdac->DMA_Handle2->XferCpltCallback = DAC_DMAConvCpltCh2; /* Set the DMA half transfer complete callback for channel2 */ hdac->DMA_Handle2->XferHalfCpltCallback = DAC_DMAHalfConvCpltCh2; /* Set the DMA error callback for channel2 */ hdac->DMA_Handle2->XferErrorCallback = DAC_DMAErrorCh2; /* Enable the selected DAC channel2 DMA request */ hdac->Instance->CR |= DAC_CR_DMAEN2; /* Case of use of channel 2 */ switch(Alignment) { case DAC_ALIGN_12B_R: /* Get DHR12R2 address */ tmpreg = (uint32_t)&hdac->Instance->DHR12R2; break; case DAC_ALIGN_12B_L: /* Get DHR12L2 address */ tmpreg = (uint32_t)&hdac->Instance->DHR12L2; break; case DAC_ALIGN_8B_R: /* Get DHR8R2 address */ tmpreg = (uint32_t)&hdac->Instance->DHR8R2; break; default: break; } } /* Enable the DMA channel */ if(Channel == DAC_CHANNEL_1) { /* Enable the DAC DMA underrun interrupt */ __HAL_DAC_ENABLE_IT(hdac, DAC_IT_DMAUDR1); /* Enable the DMA channel */ HAL_DMA_Start_IT(hdac->DMA_Handle1, (uint32_t)pData, tmpreg, Length); } else { /* Enable the DAC DMA underrun interrupt */ __HAL_DAC_ENABLE_IT(hdac, DAC_IT_DMAUDR2); /* Enable the DMA channel */ HAL_DMA_Start_IT(hdac->DMA_Handle2, (uint32_t)pData, tmpreg, Length); } /* Enable the Peripharal */ __HAL_DAC_ENABLE(hdac, Channel); /* Process Unlocked */ __HAL_UNLOCK(hdac); /* Return function status */ return HAL_OK; } 函数编写也很容易看懂。 static void DAC_Ch1_TriangleConfig(void) { /*##-1- Initialize the DAC peripheral ######################################*/ if (HAL_DAC_Init(&DacHandle) != HAL_OK) { /* Initiliazation Error */ Error_Handler(); } /*##-2- DAC channel2 Configuration #########################################*/ sConfig.DAC_Trigger = DAC_TRIGGER_T6_TRGO; sConfig.DAC_OutputBuffer = DAC_OUTPUTBUFFER_ENABLE; if (HAL_DAC_ConfigChannel(&DacHandle, &sConfig, DACx_CHANNEL) != HAL_OK) { /* Channel configuartion Error */ Error_Handler(); } /*##-3- DAC channel2 Triangle Wave generation configuration ################*/ if (HAL_DACEx_TriangleWaveGenerate(&DacHandle, DACx_CHANNEL, DAC_TRIANGLEAMPLITUDE_1023) != HAL_OK) { /* Triangle wave generation Error */ Error_Handler(); } /*##-4- Enable DAC Channel1 ################################################*/ if (HAL_DAC_Start(&DacHandle, DACx_CHANNEL) != HAL_OK) { /* Start Error */ Error_Handler(); } /*##-5- Set DAC channel1 DHR12RD register ################################################*/ if (HAL_DAC_SetValue(&DacHandle, DACx_CHANNEL, DAC_ALIGN_12B_R, 0x100) != HAL_OK) { /* Setting value Error */ Error_Handler(); } } 三角波类似,重点也是HAL_DACEx_TriangleWaveGenerate(&DacHandle, DACx_CHANNEL, DAC_TRIANGLEAMPLITUDE_1023) HAL_StatusTypeDef HAL_DACEx_TriangleWaveGenerate(DAC_HandleTypeDef* hdac, uint32_t Channel, uint32_t Amplitude) { /* Check the parameters */ assert_param(IS_DAC_CHANNEL(Channel)); assert_param(IS_DAC_LFSR_UNMASK_TRIANGLE_AMPLITUDE(Amplitude)); /* Process locked */ __HAL_LOCK(hdac); /* Change DAC state */ hdac->State = HAL_DAC_STATE_BUSY; /* Enable the selected wave generation for the selected DAC channel */ MODIFY_REG(hdac->Instance->CR, ((DAC_CR_WAVE1)|(DAC_CR_MAMP1))<<Channel, (DAC_WAVE_TRIANGLE | Amplitude) << Channel); /* Change DAC state */ hdac->State = HAL_DAC_STATE_READY; /* Process unlocked */ __HAL_UNLOCK(hdac); /* Return function status */ return HAL_OK; } 其中#define MODIFY_REG(REG, CLEARMASK, SETMASK) WRITE_REG((REG), (((READ_REG(REG)) & (~(CLEARMASK))) | (SETMASK))) 再说一下HAL的DAC驱动的基本程序及思想方法 配置DAC通道函数: HAL_StatusTypeDef HAL_DAC_ConfigChannel(DAC_HandleTypeDef* hdac, DAC_ChannelConfTypeDef* sConfig, uint32_t Channel) { uint32_t tmpreg1 = 0, tmpreg2 = 0; /* Check the DAC parameters */ assert_param(IS_DAC_TRIGGER(sConfig->DAC_Trigger)); assert_param(IS_DAC_OUTPUT_BUFFER_STATE(sConfig->DAC_OutputBuffer)); assert_param(IS_DAC_TRIGGER(sConfig->DAC_Trigger)); assert_param(IS_DAC_CHANNEL(Channel)); /* Process locked */ __HAL_LOCK(hdac); /* Change DAC state */ hdac->State = HAL_DAC_STATE_BUSY; /* Get the DAC CR value */ tmpreg1 = DAC->CR; /* Clear BOFFx, TENx, TSELx, WAVEx and MAMPx bits */ tmpreg1 &= ~(((uint32_t)(DAC_CR_MAMP1 | DAC_CR_WAVE1 | DAC_CR_TSEL1 | DAC_CR_TEN1 | DAC_CR_BOFF1)) << Channel); /* Configure for the selected DAC channel: buffer output, trigger */ /* Set TSELx and TENx bits according to DAC_Trigger value */ /* Set BOFFx bit according to DAC_OutputBuffer value */ tmpreg2 = (sConfig->DAC_Trigger | sConfig->DAC_OutputBuffer); /* Calculate CR register value depending on DAC_Channel */ tmpreg1 |= tmpreg2 << Channel; /* Write to DAC CR */ DAC->CR = tmpreg1; /* Disable wave generation */ DAC->CR &= ~(DAC_CR_WAVE1 << Channel); /* Change DAC state */ hdac->State = HAL_DAC_STATE_READY; /* Process unlocked */ __HAL_UNLOCK(hdac); /* Return function status */ return HAL_OK; } |
谢谢分享 |
谢谢分享 |
楼主有没有HAL串口篇?最近被串口搞得很闹心…… |
跟着学习一下。。。。 |
![]() |