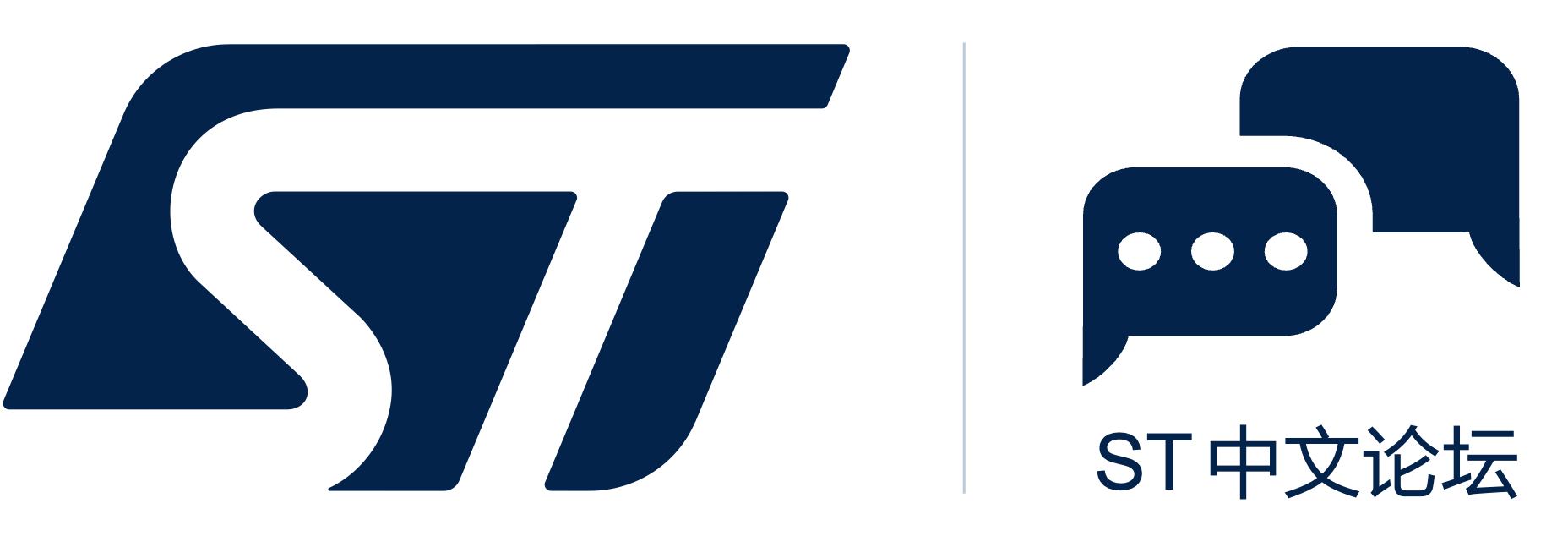
一、vector基本概念: 1、功能: vector数据结构和数组非常相似,也称为单端数组。 2、vector和普通数组的区别: 不同之处在于数组是静态空间,而vector是可以动态扩展的。动态扩展它并不是在原空间之后持续新空间,而是找更大的内存空间,然后将原数据拷贝到新空间,释放原空间。
注:上图中的push_back()和pop_back()函数分别表示往容器里面插入数据和从容器中拿走数据;begin()和end()是我们稍后会用到的迭代器 3、vector容器的迭代器是支持随机访问的迭代器。 二、vector构造函数 1、功能: 创建vector容器 2、函数原型:
代码应用: #include <iostream>#include <vector> using namespace std; //输出打印 void print(vector<int>&v) { for(vector<int>::iterator it=v.begin(); it !=v.end();it++) { cout<<*it<<" "; } cout<<endl; } //vector容器构造 void test() { vector<int>v1; for(int i =0; i<10;i++) { v1.push_back(i); } print(v1); //通过区间方式进行构造 vector<int>v2(v1.begin(),v1.end()); print(v2); //n个elem方式构造 vector<int>v3(10,100); print(v3); //拷贝构造 vector<int>v4(v3); print(v4); } int main() { test(); } 结果输出: root@txp-virtual-machine:/home/txp/test2# ./a.out0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9 100 100 100 100 100 100 100 100 100 100 100 100 100 100 100 100 100 100 100 100 三、vector赋值操作: 1、功能描述: 给vector容器进行赋值 2、函数原型:
代码应用: #include <iostream>#include <vector> using namespace std; void print(vector<int>&v) { for(vector<int>::iterator it=v.begin(); it !=v.end();it++) { cout<<*it<<" "; } cout<<endl; } void test() { vector<int>v1; for(int i=0;i<10;i++) { v1.push_back(i); } print(v1); //赋值操作 vector<int> v2; v2=v1; print(v2); //assign() vector<int>v3; v3.assign(v1.begin(),v1.end()); print(v3); //n个elem方式赋值 vector<int>v4; v4.assign(10,100); print(v4); } int main() { test(); } 结果输出: root@txp-virtual-machine:/home/txp/test2# ./a.out0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9 100 100 100 100 100 100 100 100 100 100 四、vector容量和大小: 1、功能描述: 对vector容器的容量和大小进行操作 2、函数原型:
代码应用: #include <iostream>#include <vector> using namespace std; void print(vector<int>&v) { for(vector<int>::iterator it=v.begin(); it !=v.end();it++) { cout<<*it<<" "; } cout<<endl; } void test() { vector<int>v1; for(int i=0;i<10;i++) { v1.push_back(i); } print(v1); //判断容器是否为空 if(v1.empty()) { cout<< "v1 is empty"<<endl; } else { cout<<"v1 is not empty"<<endl; cout<<"v1's capacity is : "<<v1.capacity()<<endl; cout<<"v1's size is : "<<v1.size()<<endl; } v1.resize(15); print(v1); v1.resize(15,100);//利用重载版本,可以指定默认填充,参数2,如果重新指定的比原来的长了,默认用0填充新的位置 print(v1); v1.resize(5);//如果重新指定的比原来短了,超出部分会被删除掉 print(v1); } int main() { test(); } 结果输出: root@txp-virtual-machine:/home/txp/test2# ./a.out0 1 2 3 4 5 6 7 8 9 v1 is not empty v1's capacity is : 16 v1's size is : 10 0 1 2 3 4 5 6 7 8 9 0 0 0 0 0 0 1 2 3 4 5 6 7 8 9 0 0 0 0 0 0 1 2 3 4 五、vector插入和删除: 1、功能描述:对vector容器进行插入和删除操作 2、函数原型:
#include <vector> using namespace std; void print(vector<int>&v) { for(vector<int>::iterator it=v.begin(); it !=v.end();it++) { cout<<*it<<" "; } cout<<endl; } void test() { vector<int>v1; //尾插 v1.push_back(10); v1.push_back(20); v1.push_back(30); v1.push_back(40); v1.push_back(50); print(v1); //尾删 v1.pop_back(); print(v1); //插入,第一个参数是迭代器 v1.insert(v1.begin(),100); print(v1); v1.insert(v1.begin(),2,1000); print(v1); //删除 v1.erase(v1.begin()); print(v1); //清空 v1.clear(); print(v1); } int main() { test(); } 结果输出: root@txp-virtual-machine:/home/txp/test2# ./a.out 10 20 30 40 50 10 20 30 40 100 10 20 30 40 1000 1000 100 10 20 30 40 1000 100 10 20 30 40 六、vector数据存取: 1、功能描述: 对vector中的数据进行存取操作 2、函数原型:
代码应用: #include <iostream>#include <vector> using namespace std; /*void print(vector<int>&v) { for(vector<int>::iterator it=v.begin(); it !=v.end();it++) { cout<<*it<<" "; } cout<<endl; }*/ void test() { vector<int>v1; for(int i=0;i<10;i++) { v1.push_back(i); } for(int i=0;i<10;i++) { cout<<v1<<" "; } cout<<endl; for(int i=0;i<v1.size();i++) { cout<<v1.at(i)<<" "; } cout<<endl; cout<<"the first elemt is : "<<v1.front()<<endl; cout<<"the last elemt is : "<<v1.back()<<endl; } int main() { test(); } 结果输出: root@txp-virtual-machine:/home/txp/test2# ./a.out0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9 the first elemt is : 0 the last elemt is : 9 七、vector互换容器: 1、功能描述: 实现两个容器内元素进行互换 2、函数原型: swap(vec);;//将vec与本身的元素互换 代码应用: #include <iostream>#include <vector> using namespace std; void print(vector<int>&v) { for(vector<int>::iterator it=v.begin(); it !=v.end();it++) { cout<<*it<<" "; } cout<<endl; } void test() { vector<int>v1; for(int i=0;i<10;i++) { v1.push_back(i); } print(v1); vector<int>v2; for(int i=10;i>0;i--) { v2.push_back(i); } print(v2); cout<<" after swap() "<<endl; v1.swap(v2); print(v1); print(v2); } int main() { test(); } 结果输出: root@txp-virtual-machine:/home/txp/test2# ./a.out0 1 2 3 4 5 6 7 8 9 10 9 8 7 6 5 4 3 2 1 after swap() 10 9 8 7 6 5 4 3 2 1 0 1 2 3 4 5 6 7 8 9 利用swap进行收缩内存空间 #include <iostream>#include <vector> using namespace std; /*void print(vector<int>&v) { for(vector<int>::iterator it=v.begin(); it !=v.end();it++) { cout<<*it<<" "; } cout<<endl; }*/ void test() { vector<int>v1; for(int i=0;i<10000;i++) { v1.push_back(i); } cout<<"v capacity is : "<<v1.capacity()<<endl; cout<<"v size is : "<<v1.size()<<endl; v1.resize(3); cout<<"v capacity is : "<<v1.capacity()<<endl; cout<<"v size is : "<<v1.size()<<endl; vector<int>(v1).swap(v1); cout<<"v capacity is : "<<v1.capacity()<<endl; cout<<"v size is : "<<v1.size()<<endl; } int main() { test(); } 结果输出: root@txp-virtual-machine:/home/txp/test2# ./a.outv capacity is : 16384 v size is : 10000 v capacity is : 16384 v size is : 3 v capacity is : 3 v size is : 3 八、vector预留空间: 1、功能描述: 减少vector在动态扩展容量时的扩展次数 2、函数原型: reserve(int len);//容器预留len个元素长度,预留位置不初始化,元素不可访问 代码应用: #include <iostream>#include <vector> using namespace std; /*void print(vector<int>&v) { for(vector<int>::iterator it=v.begin(); it !=v.end();it++) { cout<<*it<<" "; } cout<<endl; }*/ void test() { vector<int>v1; int num =0;//统计开辟次数 int *p = NULL; for(int i=0;i<10000;i++) { v1.push_back(i); if(p!=&v1[0]) { p = &v1[0]; num++; } } cout<<"num= "<<num<<endl; } int main() { test(); } 结果输出: root@txp-virtual-machine:/home/txp/test2# ./a.outnum= 15 |