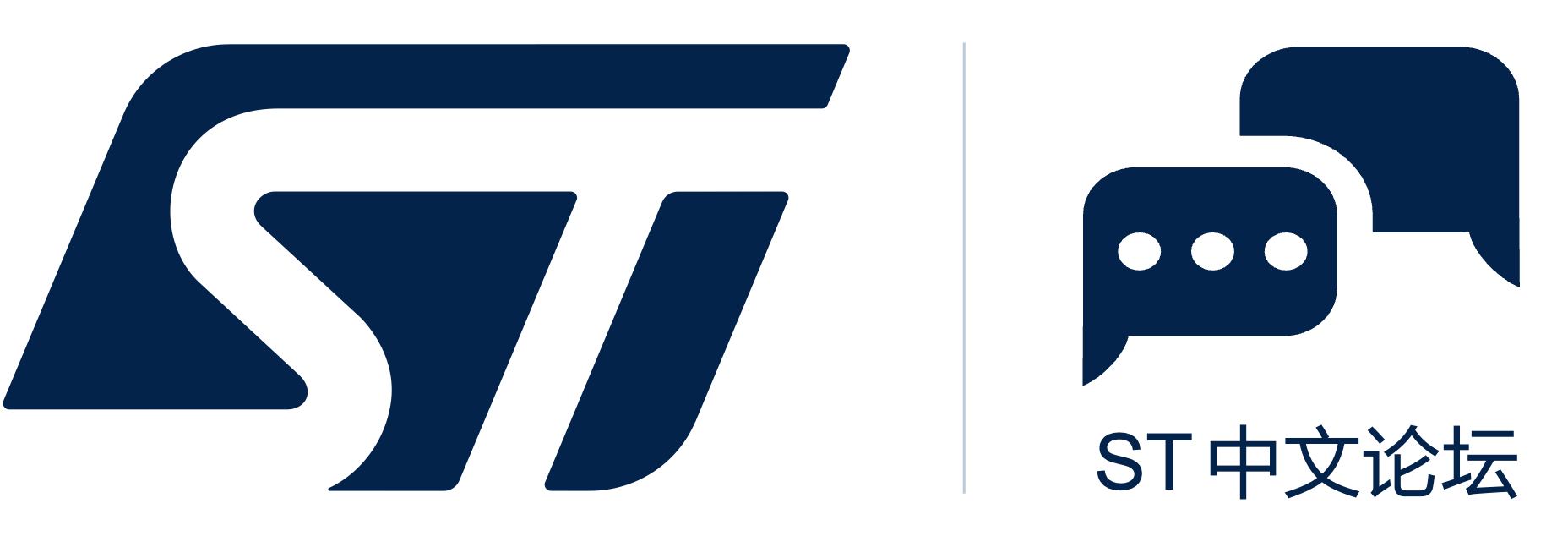
这个采用的是USART3,RE/ED接在一起,我把这个控制位置高了,就是很简单的发送一个数据给PC机,这边采用的RS485转RS232与电脑连接。但是不能正常发送数据,复位一次在串口接受界面看到00。请高手帮帮忙! /******************************************************************************* * File Name : main.c * Author : Wuhan R&D Center, Embest * Date First Issued : 08/08/2008 * Description : Main program body ********************************************************************************/ /* Includes ------------------------------------------------------------------*/ #include "stm32f10x_lib.h" #include /* Private typedef -----------------------------------------------------------*/ /* Private define ------------------------------------------------------------*/ /* Private macro -------------------------------------------------------------*/ /* Private variables ---------------------------------------------------------*/ ErrorStatus HSEStartUpStatus; /* Private function prototypes -----------------------------------------------*/ void RCC_Configuration(void); void NVIC_Configuration(void); void GPIO_Configuration(void); void USART_Configuration(void); /* Private functions ---------------------------------------------------------*/ /******************************************************************************* * Function Name : main * Description : Main program. * Input : None * Output : None * Return : None *******************************************************************************/ int main(void) { // u16 i=0; #ifdef DEBUG debug(); #endif /* Configure the system clocks */ RCC_Configuration(); /* NVIC Configuration */ NVIC_Configuration(); /* Configure the GPIOs */ GPIO_Configuration(); /* Configure the USART1 */ USART_Configuration(); // printf("\r\n Welcome to WUHAN R&D Center,Embest \r\n"); // printf("\r\n Please Input Character From Keyboard \r\n"); //while(1 ) // { // if(USART_GetFlagStatus(USART1,USART_IT_RXNE)==SET) // { // i = USART_ReceiveData(USART1); // printf(" %c",i&0xFF); /* print the input char */ // // } // } GPIOB->ODR = 0x0200; for(;;) { USART_SendData(USART3, 8); //发送一位数据 while(USART_GetFlagStatus(USART3, USART_FLAG_TXE) == RESET); GPIOA->ODR = 0x0002; } } #ifdef DEBUG /******************************************************************************* * Function Name : assert_failed * Description : Reports the name of the source file and the source line number * where the assert error has occurred. * Input : - file: pointer to the source file name * - line: assert error line source number * Output : None * Return : None *******************************************************************************/ void assert_failed(u8* file, u32 line) { /* User can add his own implementation to report the file name and line number */ printf("\n\r Wrong parameter value detected on\r\n"); printf(" file %s\r\n", file); printf(" line %d\r\n", line); /* Infinite loop */ /* while (1) { } */ } #endif /******************************************************************************* * Function Name : RCC_Configuration * Description : Configures the different system clocks. * Input : None * Output : None * Return : None *******************************************************************************/ void RCC_Configuration(void) { /* RCC system reset(for debug purpose) */ RCC_DeInit(); /* Enable HSE */ RCC_HSEConfig(RCC_HSE_ON); /* Wait till HSE is ready */ HSEStartUpStatus = RCC_WaitForHSEStartUp(); if(HSEStartUpStatus == SUCCESS) { /* HCLK = SYSCLK */ RCC_HCLKConfig(RCC_SYSCLK_Div1); /* PCLK2 = HCLK */ RCC_PCLK2Config(RCC_HCLK_Div1); /* PCLK1 = HCLK/2 */ RCC_PCLK1Config(RCC_HCLK_Div2); /* Flash 2 wait state */ FLASH_SetLatency(FLASH_Latency_2); /* Enable Prefetch Buffer */ FLASH_PrefetchBufferCmd(FLASH_PrefetchBuffer_Enable); /* PLLCLK = 8MHz * 9 = 72 MHz */ RCC_PLLConfig(RCC_PLLSource_HSE_Div1, RCC_PLLMul_9); /* Enable PLL */ RCC_PLLCmd(ENABLE); /* Wait till PLL is ready */ while(RCC_GetFlagStatus(RCC_FLAG_PLLRDY) == RESET) { } /* Select PLL as system clock source */ RCC_SYSCLKConfig(RCC_SYSCLKSource_PLLCLK); /* Wait till PLL is used as system clock source */ while(RCC_GetSYSCLKSource() != 0x08) { } } /* Enable USART1 and GPIOA clock */ RCC_APB2PeriphClockCmd( RCC_APB2Periph_GPIOB|RCC_APB2Periph_GPIOA, ENABLE); RCC_APB1PeriphClockCmd( RCC_APB1Periph_USART3,ENABLE); } /******************************************************************************* * Function Name : NVIC_Configuration * Description : Configures Vector Table base location. * Input : None * Output : None * Return : None *******************************************************************************/ void NVIC_Configuration(void) { #ifdef VECT_TAB_RAM /* Set the Vector Table base location at 0x20000000 */ NVIC_SetVectorTable(NVIC_VectTab_RAM, 0x0); #else /* VECT_TAB_FLASH */ /* Set the Vector Table base location at 0x08000000 */ NVIC_SetVectorTable(NVIC_VectTab_FLASH, 0x0); #endif } /******************************************************************************* * Function Name : GPIO_Configuration * Description : Configures the different GPIO ports. * Input : None * Output : None * Return : None *******************************************************************************/ void GPIO_Configuration(void) { GPIO_InitTypeDef GPIO_InitStructure; /* Configure PC. as Output push-pull */ GPIO_InitStructure.GPIO_Pin =GPIO_Pin_0|GPIO_Pin_1|GPIO_Pin_4|GPIO_Pin_5|GPIO_Pin_6|GPIO_Pin_7|GPIO_Pin_11|GPIO_Pin_12; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;//输出 GPIO_Init(GPIOA, &GPIO_InitStructure); GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; GPIO_Init(GPIOB, &GPIO_InitStructure); /* Configure USART1 Tx (PB.10) as alternate function push-pull */ GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_Init(GPIOB, &GPIO_InitStructure); /* Configure USART1 Rx (PAB.11) as input floating */ GPIO_InitStructure.GPIO_Pin = GPIO_Pin_11; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_Init(GPIOB, &GPIO_InitStructure); } /******************************************************************************* * Function Name : USART_Configuration * Description : Configures the USART1. * Input : None * Output : None * Return : None *******************************************************************************/ void USART_Configuration(void) { USART_InitTypeDef USART_InitStructure; USART_ClockInitTypeDef USART_ClockInitStructure; /* USART1 configuration ----------------------------------------------- -------*/ /* USART1 configured as follow: - BaudRate = 115200 baud - Word Length = 8 Bits - One Stop Bit - No parity - Hardware flow control disabled (RTS and CTS signals) - Receive and transmit enabled - USART Clock disabled - USART CPOL: Clock is active low - USART CPHA: Data is captured on the middle - USART LastBit: The clock pulse of the last data bit is not output to the SCLK pin */ USART_ClockInitStructure.USART_Clock = USART_Clock_Disable; USART_ClockInitStructure.USART_CPOL = USART_CPOL_Low; USART_ClockInitStructure.USART_CPHA = USART_CPHA_2Edge; USART_ClockInitStructure.USART_LastBit = USART_LastBit_Disable; /* Configure the USART1 synchronous paramters */ USART_ClockInit(USART3, &USART_ClockInitStructure); USART_InitStructure.USART_BaudRate = 9600; USART_InitStructure.USART_WordLength = USART_WordLength_8b; USART_InitStructure.USART_StopBits = USART_StopBits_1; USART_InitStructure.USART_Parity = USART_Parity_No ; USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None; USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx; /* Configure USART1 basic and asynchronous paramters */ USART_Init(USART3, &USART_InitStructure); /* Enable USART1 */ USART_Cmd(USART3, ENABLE); } /******************************************************************************* * Function Name : fputc * Description : Retargets the C library printf function to the USART. * Input : None * Output : None * Return : None *******************************************************************************/ int fputc(int ch, FILE *f) { /* Place your implementation of fputc here */ /* e.g. write a character to the USART */ USART_SendData(USART3, (u8) ch); /* Loop until the end of transmission */ while(USART_GetFlagStatus(USART3, USART_FLAG_TC) == RESET) { } return ch; } /******************* (C) COPYRIGHT 2007 STMicroelectronics *****END OF FILE****/ /******************************************************************************* * File Name : main.c * Author : Wuhan R&D Center, Embest * Date First Issued : 08/08/2008 * Description : Main program body ********************************************************************************/ /* Includes ------------------------------------------------------------------*/ #include "stm32f10x_lib.h" #include /* Private typedef -----------------------------------------------------------*/ /* Private define ------------------------------------------------------------*/ /* Private macro -------------------------------------------------------------*/ /* Private variables ---------------------------------------------------------*/ ErrorStatus HSEStartUpStatus; /* Private function prototypes -----------------------------------------------*/ void RCC_Configuration(void); void NVIC_Configuration(void); void GPIO_Configuration(void); void USART_Configuration(void); /* Private functions ---------------------------------------------------------*/ /******************************************************************************* * Function Name : main * Description : Main program. * Input : None * Output : None * Return : None *******************************************************************************/ int main(void) { // u16 i=0; #ifdef DEBUG debug(); #endif /* Configure the system clocks */ RCC_Configuration(); /* NVIC Configuration */ NVIC_Configuration(); /* Configure the GPIOs */ GPIO_Configuration(); /* Configure the USART1 */ USART_Configuration(); // printf("\r\n Welcome to WUHAN R&D Center,Embest \r\n"); // printf("\r\n Please Input Character From Keyboard \r\n"); //while(1 ) // { // if(USART_GetFlagStatus(USART1,USART_IT_RXNE)==SET) // { // i = USART_ReceiveData(USART1); // printf(" %c",i&0xFF); /* print the input char */ // // } // } GPIOB->ODR = 0x0200; for(;;) { USART_SendData(USART3, 8); //发送一位数据 while(USART_GetFlagStatus(USART3, USART_FLAG_TXE) == RESET); GPIOA->ODR = 0x0002; } } #ifdef DEBUG /******************************************************************************* * Function Name : assert_failed * Description : Reports the name of the source file and the source line number * where the assert error has occurred. * Input : - file: pointer to the source file name * - line: assert error line source number * Output : None * Return : None *******************************************************************************/ void assert_failed(u8* file, u32 line) { /* User can add his own implementation to report the file name and line number */ printf("\n\r Wrong parameter value detected on\r\n"); printf(" file %s\r\n", file); printf(" line %d\r\n", line); /* Infinite loop */ /* while (1) { } */ } #endif /******************************************************************************* * Function Name : RCC_Configuration * Description : Configures the different system clocks. * Input : None * Output : None * Return : None *******************************************************************************/ void RCC_Configuration(void) { /* RCC system reset(for debug purpose) */ RCC_DeInit(); /* Enable HSE */ RCC_HSEConfig(RCC_HSE_ON); /* Wait till HSE is ready */ HSEStartUpStatus = RCC_WaitForHSEStartUp(); if(HSEStartUpStatus == SUCCESS) { /* HCLK = SYSCLK */ RCC_HCLKConfig(RCC_SYSCLK_Div1); /* PCLK2 = HCLK */ RCC_PCLK2Config(RCC_HCLK_Div1); /* PCLK1 = HCLK/2 */ RCC_PCLK1Config(RCC_HCLK_Div2); /* Flash 2 wait state */ FLASH_SetLatency(FLASH_Latency_2); /* Enable Prefetch Buffer */ FLASH_PrefetchBufferCmd(FLASH_PrefetchBuffer_Enable); /* PLLCLK = 8MHz * 9 = 72 MHz */ RCC_PLLConfig(RCC_PLLSource_HSE_Div1, RCC_PLLMul_9); /* Enable PLL */ RCC_PLLCmd(ENABLE); /* Wait till PLL is ready */ while(RCC_GetFlagStatus(RCC_FLAG_PLLRDY) == RESET) { } /* Select PLL as system clock source */ RCC_SYSCLKConfig(RCC_SYSCLKSource_PLLCLK); /* Wait till PLL is used as system clock source */ while(RCC_GetSYSCLKSource() != 0x08) { } } /* Enable USART1 and GPIOA clock */ RCC_APB2PeriphClockCmd( RCC_APB2Periph_GPIOB|RCC_APB2Periph_GPIOA, ENABLE); RCC_APB1PeriphClockCmd( RCC_APB1Periph_USART3,ENABLE); } /******************************************************************************* * Function Name : NVIC_Configuration * Description : Configures Vector Table base location. * Input : None * Output : None * Return : None *******************************************************************************/ void NVIC_Configuration(void) { #ifdef VECT_TAB_RAM /* Set the Vector Table base location at 0x20000000 */ NVIC_SetVectorTable(NVIC_VectTab_RAM, 0x0); #else /* VECT_TAB_FLASH */ /* Set the Vector Table base location at 0x08000000 */ NVIC_SetVectorTable(NVIC_VectTab_FLASH, 0x0); #endif } /******************************************************************************* * Function Name : GPIO_Configuration * Description : Configures the different GPIO ports. * Input : None * Output : None * Return : None *******************************************************************************/ void GPIO_Configuration(void) { GPIO_InitTypeDef GPIO_InitStructure; /* Configure PC. as Output push-pull */ GPIO_InitStructure.GPIO_Pin =GPIO_Pin_0|GPIO_Pin_1|GPIO_Pin_4|GPIO_Pin_5|GPIO_Pin_6|GPIO_Pin_7|GPIO_Pin_11|GPIO_Pin_12; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;//输出 GPIO_Init(GPIOA, &GPIO_InitStructure); GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; GPIO_Init(GPIOB, &GPIO_InitStructure); /* Configure USART1 Tx (PB.10) as alternate function push-pull */ GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_Init(GPIOB, &GPIO_InitStructure); /* Configure USART1 Rx (PAB.11) as input floating */ GPIO_InitStructure.GPIO_Pin = GPIO_Pin_11; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_Init(GPIOB, &GPIO_InitStructure); } /******************************************************************************* * Function Name : USART_Configuration * Description : Configures the USART1. * Input : None * Output : None * Return : None *******************************************************************************/ void USART_Configuration(void) { USART_InitTypeDef USART_InitStructure; USART_ClockInitTypeDef USART_ClockInitStructure; /* USART1 configuration ----------------------------------------------- -------*/ /* USART1 configured as follow: - BaudRate = 115200 baud - Word Length = 8 Bits - One Stop Bit - No parity - Hardware flow control disabled (RTS and CTS signals) - Receive and transmit enabled - USART Clock disabled - USART CPOL: Clock is active low - USART CPHA: Data is captured on the middle - USART LastBit: The clock pulse of the last data bit is not output to the SCLK pin */ USART_ClockInitStructure.USART_Clock = USART_Clock_Disable; USART_ClockInitStructure.USART_CPOL = USART_CPOL_Low; USART_ClockInitStructure.USART_CPHA = USART_CPHA_2Edge; USART_ClockInitStructure.USART_LastBit = USART_LastBit_Disable; /* Configure the USART1 synchronous paramters */ USART_ClockInit(USART3, &USART_ClockInitStructure); USART_InitStructure.USART_BaudRate = 9600; USART_InitStructure.USART_WordLength = USART_WordLength_8b; USART_InitStructure.USART_StopBits = USART_StopBits_1; USART_InitStructure.USART_Parity = USART_Parity_No ; USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None; USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx; /* Configure USART1 basic and asynchronous paramters */ USART_Init(USART3, &USART_InitStructure); /* Enable USART1 */ USART_Cmd(USART3, ENABLE); } /******************************************************************************* * Function Name : fputc * Description : Retargets the C library printf function to the USART. * Input : None * Output : None * Return : None *******************************************************************************/ int fputc(int ch, FILE *f) { /* Place your implementation of fputc here */ /* e.g. write a character to the USART */ USART_SendData(USART3, (u8) ch); /* Loop until the end of transmission */ while(USART_GetFlagStatus(USART3, USART_FLAG_TC) == RESET) { } return ch; } /******************* (C) COPYRIGHT 2007 STMicroelectronics *****END OF FILE****/ |
main.txt
下载9.28 KB, 下载次数: 16, 下载积分: ST金币 -1
【MCU实战经验】基于STM32F103C8T6的hart总线调试器设计
求教STM32F103进入STOP模式后用外部中断唤醒的问题
基于STM32F103RCT6的无源蜂鸣器音乐播放(生日快乐歌)
STM32F103c8t6有没有DAC 功能?
STM32F103x中文数据手册
新手求教,为何在我电脑上找不到STM32F1Xx.h文件
金龙107例程汇总(STM32F107)
万利STM32F107VC 原理图
STM32F103 ADC多通道检测必须要DMA吗?
【官方例程】STM32F107以太网官方例程
RE:高手帮我看看STM32F101的RS485通信
RE:高手帮我看看STM32F101的RS485通信
RE:高手帮我看看STM32F101的RS485通信
1.RE/ED端在转换发送接收时一定要加足够的延时,因为485IC比不了我们的STM32F101快.即使没有改变也最好加点延时
2.还有你的IO口: GPIOB->ODR = 0x0200;和GPIOA->ODR = 0x0002;是干什么用的?你是否有控制RE/ED端为发送.
RE:高手帮我看看STM32F101的RS485通信
Available only on devices with a Flash memory density equal or higher than 64 Kbytes.
我选的是STM32F101C6。
我换了个UASRT2试了试可用发送了。