基础测评参考:https://shequ.stmicroelectronics.cn/thread-643647-1-1.html
在FOC算法中,需要计算电机的角度和位置,这通常涉及到正弦和余弦函数的计算。这些计算可以帮助确定电机的最佳控制策略,以确保电机能够根据控制信号高效地转换电能到机械能。在实际应用中,由于直接计算三角函数可能较为耗时,所以,本次测试使用数学三角函数计算,来对比运行速度。
以Sin()为例:
在main.c中添加代码
添加头文件#include <math.h>
定义变量
uint32_t StartTimeStamp = 0;//记录运算前的时间戳
uint32_t StopTimeStamp = 0;//记录运算后前的时间戳
uint16_t RandomAngle = 0;//生成随机的角度用于计算,但发现随机的角度计算的时间差异较大,没法比较,所以程序里还是用固定值来作计算比较
uint32_t random32bit;
double Result;//存放Sin()计算结果
在原按键响应程序中添加以下代码:
HAL_RNG_GenerateRandomNumber(&hrng, &random32bit);
RandomAngle = 45;//random32bit % 360;
StartTimeStamp = __HAL_TIM_GET_COUNTER(&htim2);
Result = sin(RandomAngle*(3.1415926)/180);
StopTimeStamp = __HAL_TIM_GET_COUNTER(&htim2);
printf("\n\r");
sprintf(DispStr, "Random angle is: %d .\n\r", RandomAngle);
printf(DispStr);
sprintf(DispStr, "The Start timestamp is: %d .\n\r", StartTimeStamp);
printf(DispStr);
sprintf(DispStr, "The Stop timestamp is: %d .\n\r", StopTimeStamp);
printf(DispStr);
sprintf(DispStr, "The result of calculating sin() is: %f .\n\r", Result);
printf(DispStr);
sprintf(DispStr, "The time consumed by the calculation is: %d us.\n\r ", (StopTimeStamp - StartTimeStamp));
printf(DispStr);
保持上个工程的参数,主频32M,未开启ICACHE,运行结果,94us左右
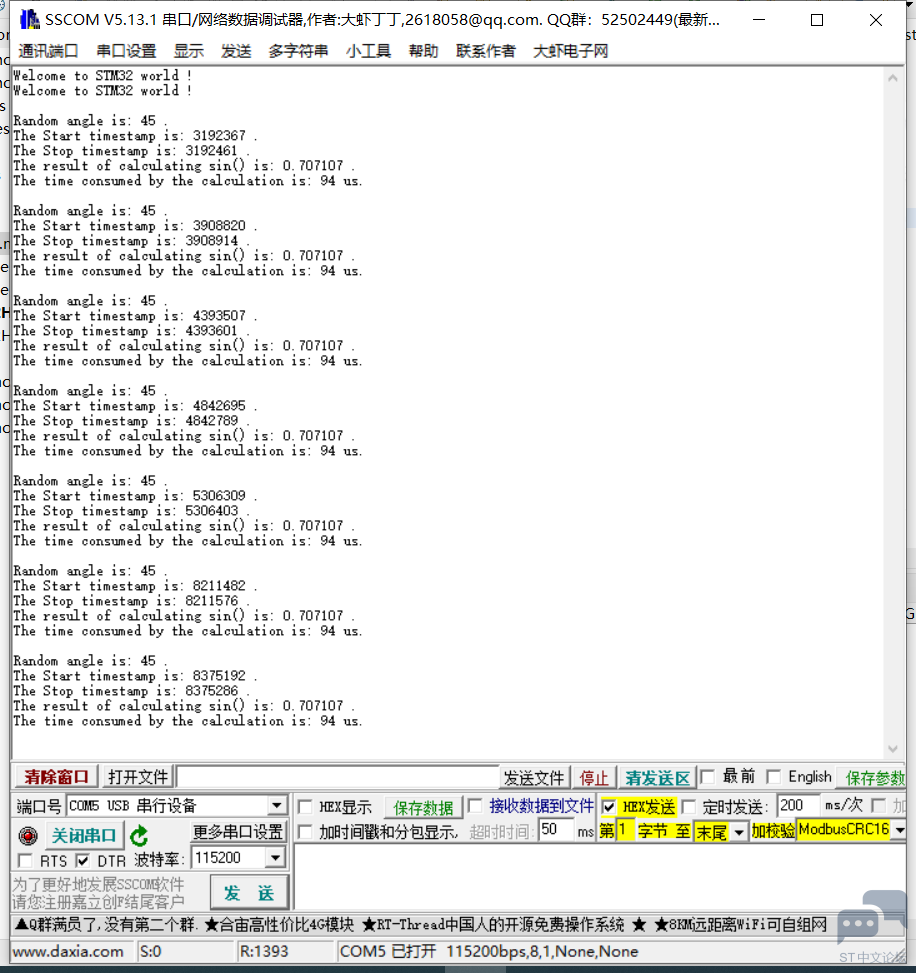
修改主频到250MHz
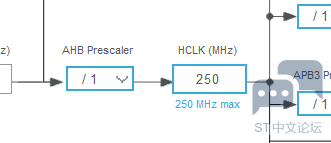
定时器也同步修改一下,保证1us的时基
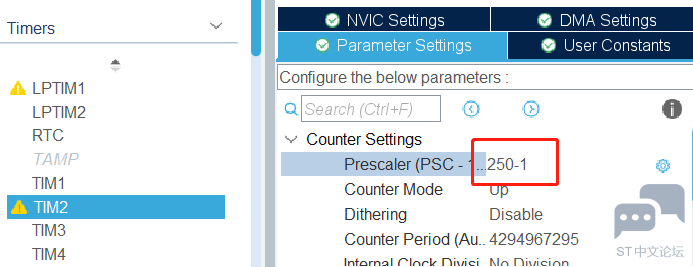
主频250M,未开启ICACHE,运行结果,15us左右
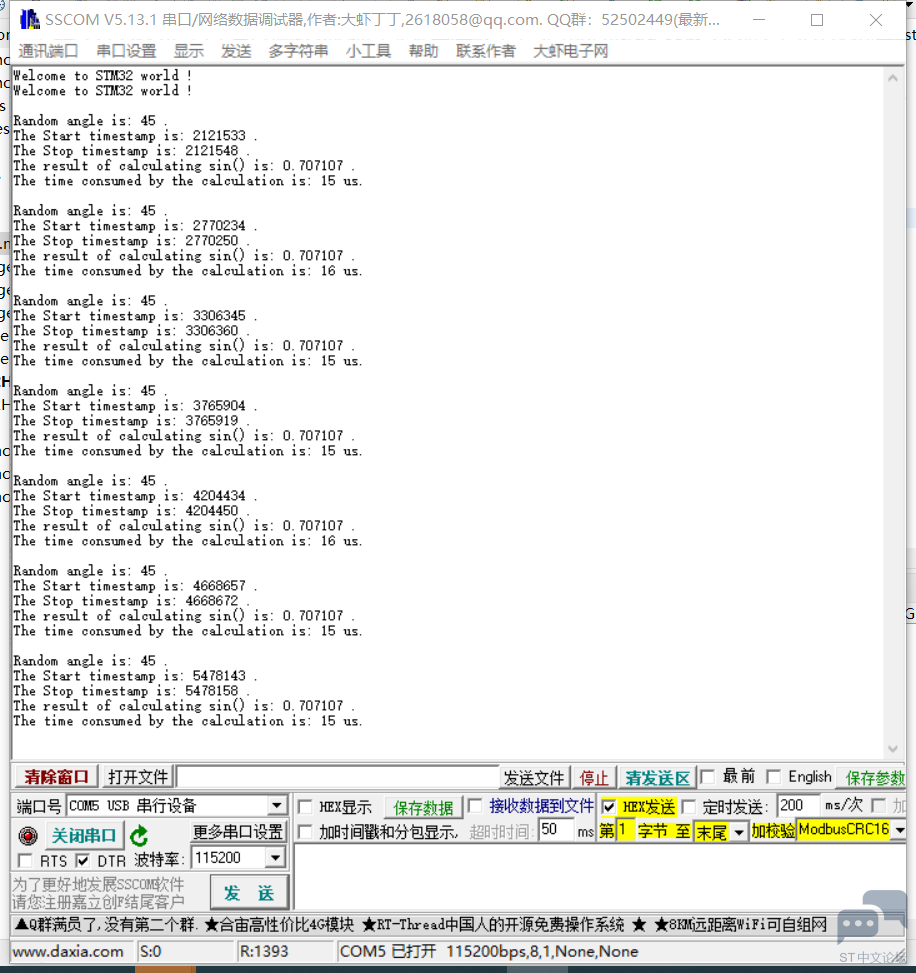
开启ICACHE,1-way,运行结果,10us左右

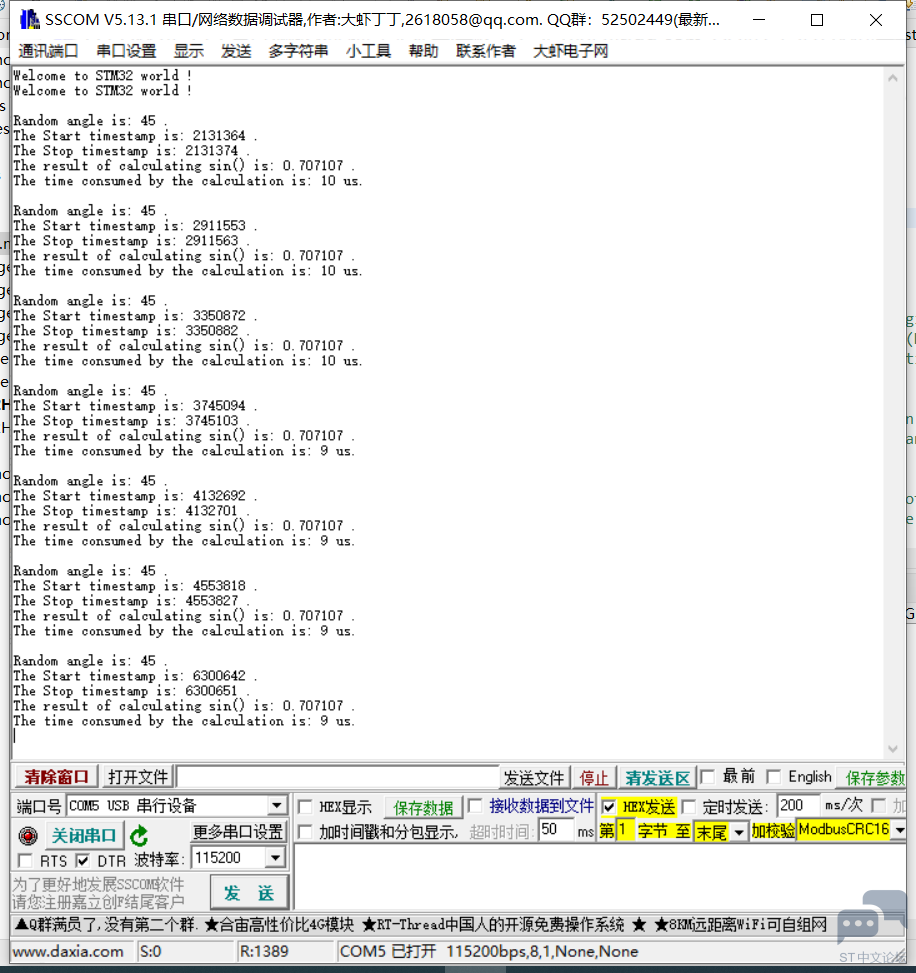
开启ICACHE,2-ways,运行结果,9us左右,好像又快了一丢丢。

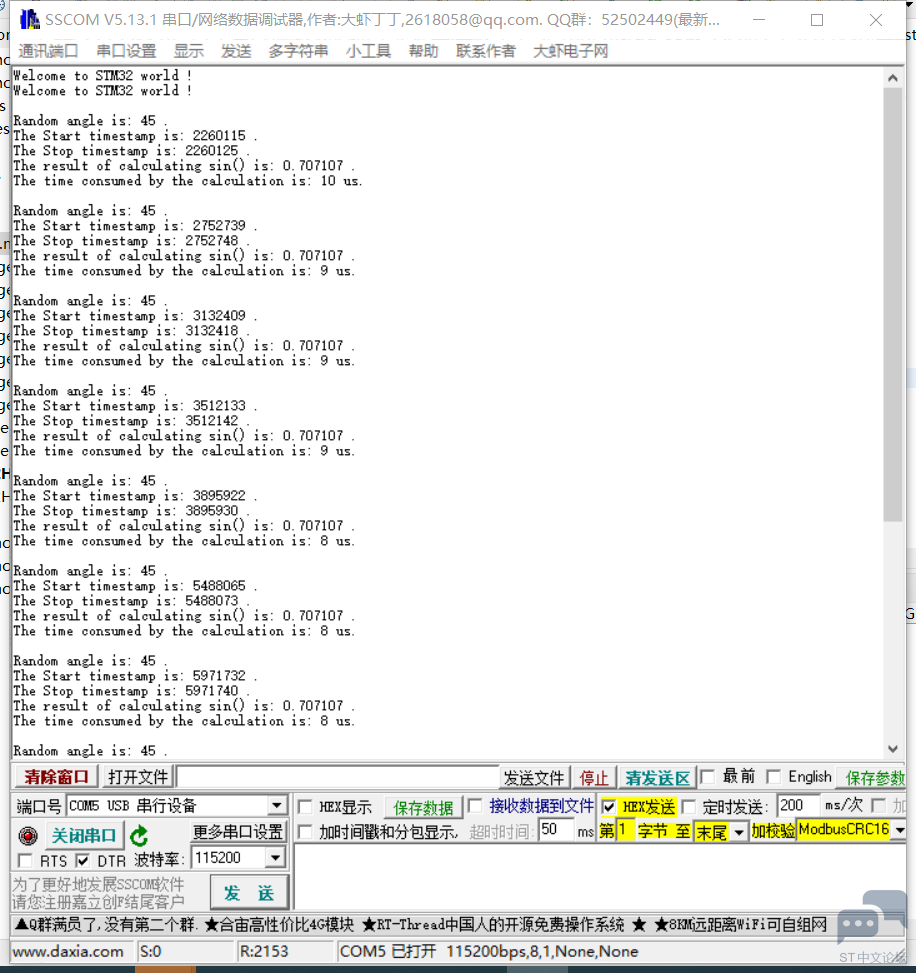
对于ICACHE的用法,我还不是太清楚,目前只会要把Cube来配置使用,按照测试,这个性能至少提升了30%以上,针对一些高速运算、控制方面,这个性能杠杠的。
修改代码全貌
/* Private includes ----------------------------------------------------------*/
/* USER CODE BEGIN Includes */
#include <math.h>
/* USER CODE END Includes */
/* Private typedef -----------------------------------------------------------*/
/* USER CODE BEGIN PTD */
/* USER CODE END PTD */
/* Private define ------------------------------------------------------------*/
/* USER CODE BEGIN PD */
/* USER CODE END PD */
/* Private macro -------------------------------------------------------------*/
/* USER CODE BEGIN PM */
/* USER CODE END PM */
/* Private variables ---------------------------------------------------------*/
COM_InitTypeDef BspCOMInit;
__IO uint32_t BspButtonState = BUTTON_RELEASED;
RNG_HandleTypeDef hrng;
TIM_HandleTypeDef htim2;
/* USER CODE BEGIN PV */
uint32_t StartTimeStamp = 0;
uint32_t StopTimeStamp = 0;
uint16_t RandomAngle = 0;
uint32_t random32bit;
double Result;
/* USER CODE END PV */
/* Private function prototypes -----------------------------------------------*/
void SystemClock_Config(void);
static void MX_GPIO_Init(void);
static void MX_TIM2_Init(void);
static void MX_RNG_Init(void);
static void MX_ICACHE_Init(void);
/* USER CODE BEGIN PFP */
/* USER CODE END PFP */
/* Private user code ---------------------------------------------------------*/
/* USER CODE BEGIN 0 */
/* USER CODE END 0 */
/**
* @brief The application entry point.
* @retval int
*/
int main(void)
{
/* USER CODE BEGIN 1 */
char DispStr[64];
/* USER CODE END 1 */
/* MCU Configuration--------------------------------------------------------*/
/* Reset of all peripherals, Initializes the Flash interface and the Systick. */
HAL_Init();
/* USER CODE BEGIN Init */
/* USER CODE END Init */
/* Configure the system clock */
SystemClock_Config();
/* USER CODE BEGIN SysInit */
/* USER CODE END SysInit */
/* Initialize all configured peripherals */
MX_GPIO_Init();
MX_TIM2_Init();
MX_RNG_Init();
MX_ICACHE_Init();
/* USER CODE BEGIN 2 */
/* USER CODE END 2 */
/* Initialize leds */
BSP_LED_Init(LED_GREEN);
/* Initialize USER push-button, will be used to trigger an interrupt each time it's pressed.*/
BSP_PB_Init(BUTTON_USER, BUTTON_MODE_EXTI);
/* Initialize COM1 port (115200, 8 bits (7-bit data + 1 stop bit), no parity */
BspCOMInit.BaudRate = 115200;
BspCOMInit.WordLength = COM_WORDLENGTH_8B;
BspCOMInit.StopBits = COM_STOPBITS_1;
BspCOMInit.Parity = COM_PARITY_NONE;
BspCOMInit.HwFlowCtl = COM_HWCONTROL_NONE;
if (BSP_COM_Init(COM1, &BspCOMInit) != BSP_ERROR_NONE)
{
Error_Handler();
}
/* USER CODE BEGIN BSP */
/* -- Sample board code to send message over COM1 port ---- */
printf("Welcome to STM32 world !\n\r");
/* -- Sample board code to switch on leds ---- */
BSP_LED_On(LED_GREEN);
HAL_TIM_Base_Start(&htim2);
/* USER CODE END BSP */
/* Infinite loop */
/* USER CODE BEGIN WHILE */
while (1)
{
/* -- Sample board code for User push-button in interrupt mode ---- */
if (BspButtonState == BUTTON_PRESSED)
{
/* Update button state */
BspButtonState = BUTTON_RELEASED;
/* -- Sample board code to toggle leds ---- */
BSP_LED_Toggle(LED_GREEN);
/* ..... Perform your action ..... */
HAL_RNG_GenerateRandomNumber(&hrng, &random32bit);
RandomAngle = 45;//random32bit % 360;
StartTimeStamp = __HAL_TIM_GET_COUNTER(&htim2);
Result = sin(RandomAngle*(3.1415926)/180);
StopTimeStamp = __HAL_TIM_GET_COUNTER(&htim2);
printf("\n\r");
sprintf(DispStr, "Random angle is: %d .\n\r", RandomAngle);
printf(DispStr);
sprintf(DispStr, "The Start timestamp is: %d .\n\r", StartTimeStamp);
printf(DispStr);
sprintf(DispStr, "The Stop timestamp is: %d .\n\r", StopTimeStamp);
printf(DispStr);
sprintf(DispStr, "The result of calculating sin() is: %f .\n\r", Result);
printf(DispStr);
sprintf(DispStr, "The time consumed by the calculation is: %d .\n\r ", (StopTimeStamp - StartTimeStamp));
printf(DispStr);
//sprintf(DispStr, "Random angle is: %d .\n\r The Start timestamp is: %d .\n\r The Start timestamp is: %d .\n\r The result of calculating sin() is: %f .\n\r The time consumed by the calculation is: %d .\n\r ", RandomAngle, StartTimeStamp, StopTimeStamp, a, (StopTimeStamp - StartTimeStamp));
}
/* USER CODE END WHILE */
/* USER CODE BEGIN 3 */
}
/* USER CODE END 3 */
}
可以在无刷电机控制中使用